How-To Guides & Tutorials
Test-Driven Development (TDD): A Practical Introduction to Writing Tests First
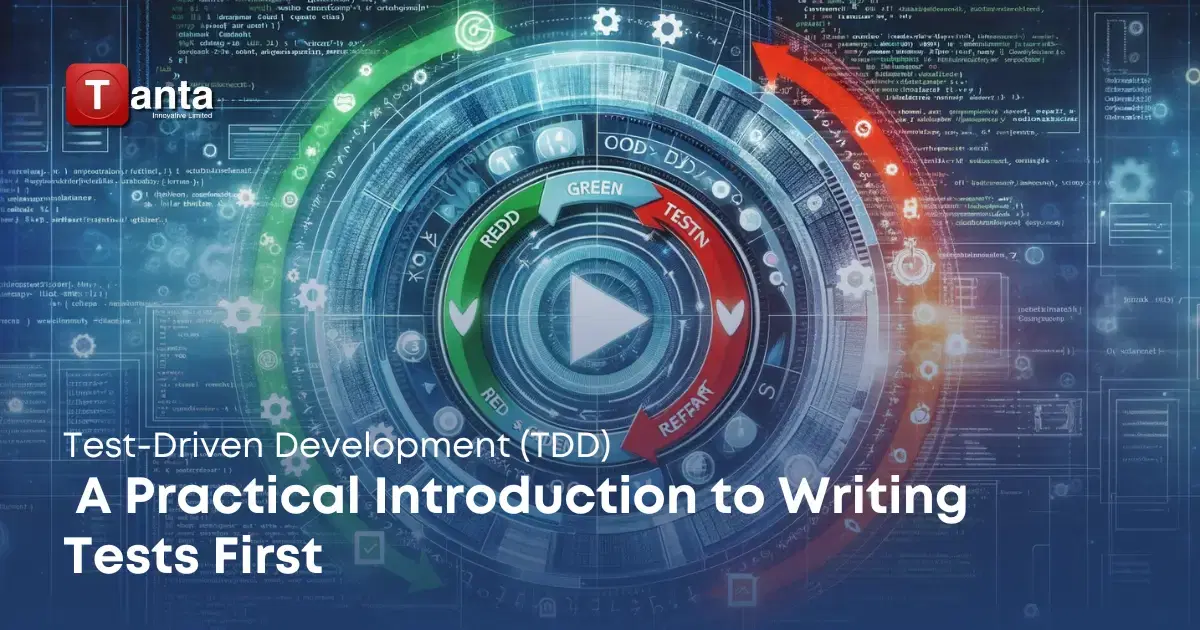
Introduction
My experience as a developer has shown me, in a very direct way, that the software development landscape undergoes constant changes, forcing us to find the most effective ways to provide high-quality solutions and to do it in a less time-consuming way. Through the adoption of Test-Driven Development (TDD), one methodology that has made a great difference in terms of how I code is TDD. It's a simple yet powerful concept: first of all, write your test cases first, then write your code. The method is not only there to make sure that the functioning of the code is at par with the requirement from the beginning; it also develops the analytical ability of the programmers for the development process.
Let's say I could boil the result of what TDD means down to three points – decreasing amount of bugs, improving the quality of code, and creating an environment where changes can be made confidently. It obliges us to consider the things about the code that is responsible for it working and makes it look as good as possible thereby, making our work more efficient. Speaking of tests, TDD precedes the development of a test case, so the code always has specific purposes and meets the already identified criteria every time it is included in the codebase.
The adoption of TDD as the practice of our team has greatly changed my life and the experience of working with me. It enhances the correctness of the software, reduces the amount of time spent on development, and reveals more about our codebase deepness. While travelling through TDD with you, I will tell you the practical application of it right from the benefits it also brings. Whether you have a couple of years of experience in the field or you are still a rookie, moving through the rungs of TDD will help you grasp the art of software development.
Understanding Test-Driven Development (TDD)
To put it simply, Test-Driven Development (TDD) is the software development process which, at the same time, follows a non-traditional stepwise pattern. With TDD, we begin by writing tests for the functionality or features we intend to build even before writing the necessary source code. These tests shall be built to fail in the beginning which will set a a specific goal for what is needed to be accomplished. The "Red, Green, Refactor" Cycle. The TDD process is often summarized by the "Red, Green, Refactor" cycle:
- Red: We write a test for a new feature or improvement, and run it! Since the feature is yet to be implemented, the test does not pass. That is the "RED" phase, when 'red' is the indicator, meaning the test did not pass.
- Green: Next we will write the minimum amount of code to make our test pass like say a class or method. Meaning the code is not perfect or completed, but it fulfils the conditions of the test. When the test passes, we are in the green phase.
- Refactor: Lastly, we analyze the code we have written and ask ourselves if it can be improved. If yes, we reengine the line of code and make sure that our test still works fine after the modifications. This is the "Refactor phase."
Code Example: The Clean Green and Refactor Cycle
Let's illustrate the TDD cycle with a simple example in Python. Suppose we want to create a function that adds two numbers:
import unittest
class TestCalculator(unittest.TestCase):
def test_add_two_numbers(self):
self.assertEqual(add(2, 3), 5)
def add(x, y):
return x + y
if __name__ == '__main__':
unittest.main()
- Red: We start by writing a test for our add function. At this point, the add function doesn't exist, so running the test will fail.
- Green: We then write the simplest possible implementation of the add function to make the test pass:
def add(x, y): return x + y
- Refactor: In this stage, we check if the code has any room for improvement. This illustration might not involve much refactoring, but in more complex cases it is fundamental for writing clean, neat and efficient code.
Expanding the Example
To further demonstrate TDD, we can add more test cases to cover edge cases:
class TestCalculator(unittest.TestCase):
def test_add_two_numbers(self):
self.assertEqual(add(2, 3), 5)
def test_add_negative_numbers(self):
self.assertEqual(add(-2, -3), -5)
def test_add_zero(self):
self.assertEqual(add(0, 0), 0)
By following this cycle, we ensure that our code is always tested and that we're continuously improving its quality. In the next sections, we'll explore the benefits of TDD and how to implement it in more detail.
The Benefits of TDD
Using Test-Driven Development (TDD) helps to offer several benefits, which in effect can highly enhance both the quality and maintainability of your code production. Here are some of the key benefits: Here are some of the key benefits: Improved Code Quality One of TDD's important features is that each code is written to pass certain tests purpose. This in turn ensures that the code base is always validated against the requirements as bugs and errors are rare trends that are present. Furthermore, as the test itself was first written, this can ensure the developers write more, thus the code focuses on modular or moving parts of the system. Better Design TDD encourages developers to think about the design and architecture of their code from the beginning. By focusing on writing tests first, developers are forced to consider how their code will be used and how it will interact with other parts of the system. This leads to a more thoughtful and deliberate design process, resulting in cleaner and more maintainable code. Faster Feedback Among the primary advantages of TDD is the rapid feedback it gives when it is applied. First, developing test cases is executed before the code, allowing early tracking and resolution of issues at the start of development. This also puts forward the justification for using agile methods of the project as it significantly accelerates the process of development which as a result reduces time and the effort spent on debugging and fixing bugs later on. Living Documentation Tests created using TDD stand as additional documentation for the code base, therefore, reducing the need for documentation files. It is demonstrated that the programmers write codes specifically for new developers to facilitate their understanding of the systems. This document, which is a dynamic and real-time one, consists of a sequence of changes over the codebase.
Implementing TDD: Part by Part Stape
Now that we looked at the advantages of test-driven development, let’s move to a hands-on practical guide that explains how to apply this methodology to your workflow.
- Step 1: Start with the Testing Kick things off with a test for the smallest feature containing the improvement that you have planned. This test has to be straightforward and put its emphasis on a particular aspect of the functionality.
- Step 2: Run the test After modifying the code, run the test to make sure that it ultimately fails. It is a very important stage, which verifies to the fact that the test is valid and that the missing feature is indeed not working or functioning faulty.
- Step 3: Write the Code Write the minimum amount of code so that it passes the test. Now you don't need to worry about writing perfect or complete code because the key is to pass the tests.
- Step 4: Optimize the Code Upon the test completion, take some time to clean up the code. See if you can do anything about the structure, readability, or speed of the test while at the same time keeping it within the standards.
- Step 5: Repeat Do this sequentially, adding more tests and making the system more reliable as you go. As you move through one loop, you will be building comprehensive tests which will also be the security net for the next patches.
Through this process and using the concept of TDD, you can make sure that your code's quality, stability, trustworthiness, and throughput get better. Coming up, we will examine some problems there may be in TDD and why it matters to be able to overcome them.
Overcoming TDD Challenges
Initial Learning Curve
Learning TDD might seem uncertain at the beginning but it is worth a try. It’s a swap of mindset where you start by writing tests first instead of code. To familiarize yourself with the TDD you've got to try something easy to start with and then increase to moderate challenges. There are also lots of tools and courses on the internet you can use to familiarize yourself with a TDD style of programming which is widely applicable to almost any programming language.
"Where to Start?"
Faced with TDD? Don’t try implementing the biggest one; begin with the simplest feature or improvement. In the same way, you'd do TDD, to test the specific part you want to test, you need to write up the test, and then write the code to pass that test. The step-by-step guidance would rather break down the whole process into small achievable pieces. So you have always got a small objective to work on. This would make your project plan easily implementable and successful.
TDD in Practice: Case Studies
One of the biggest advantages of using test-driven development (TDD) in real-world projects can be noticed in notable improvement of code quality and the project development process. Here are some case studies where TDD has been successfully implemented:
Industrial Case Study on TDD Effectiveness
A working paper investigated the performance of TDD within a real sector as evidenced by the monitoring of selected 3 middle-range projects. This research showed that TDD had resulted in there being a more methodical way of testing and development. This resulted in cleaner work and a faster development process. Read more
TDD at Microsoft and IBM
Test-driven development (TDD) case studies were conducted among teams of developers at Microsoft and IBM that adopted this approach and the lower post-release defect density proved it with real written words. The defect density of the products developed with TDD decreased between 40% and 90% relative to similar projects that did not use the TDD practice. This highlights the impact of TDD on improving software quality before release. Read more
Evaluating TDD Efficacy in Different Environments
Another research tried to measure the efficiency of the TDD used in software development on two different environments at Microsoft (Windows and MSN divisions). The result shows that code quality for TDD projects is much higher by around 40% than the number of projects that do not use TDD. Read more
TDD in Day-to-day Working Java Projects
The Apache Tomcat enterprise-level Java web application server project depicts one of the best cases when the TDD is widely used within the Java ecosystem. The Apache Tomcat team has been following the red beer approach strictly of TDD by writing tests before executing a feature. This has led to a credible and well-built web server and a servlet container.
The described case studies are an example that TDD is efficient in different contexts: from large-scale projects, corporations or open-source projects. Adopting TDD can contribute significantly to code quality improvement, the reduction of bugs and the streamlining development process.
SEE: Testing Tools for Popular Programming Languages
Related Posts
No related posts found.