How-To Guides & Tutorials
How to Integrate Intercom in Your Next.js 14 Project

Intercom has become an indispensable tool for businesses looking to enhance their customer communication experience. Its real-time messaging capability allows you to engage with your customers directly and personally. If you're working with the latest Next.js 14, integrating Intercom into your project can supercharge your customer interactions. Here's a definitive guide crafted for tech enthusiasts who demand the best from their tools.
Prerequisites
- A Next.js 14 project already up and running.
- An active Intercom account and access to your unique app ID.
Step-by-Step Integration Guide
Integrating Intercom with Next.js 14 involves creating a dedicated component and embedding it within your layout structure. This process ensures that Intercom's scripts load efficiently, aligning with Next.js 14’s advanced script management capabilities.
Step 1: Creating the `IntercomClientComponent
First, we'll create a component dedicated to initializing Intercom in your application. Follow these steps:
- Navigate to your components directory and create a new file named
IntercomClientComponent.tsx.
- Copy and paste the following TypeScript code into your new component file:
// src/components/IntercomClientComponent.tsx 'use client'; import { useEffect } from "react"; const IntercomClientComponent: React.FC = () => { useEffect(() => { window.intercomSettings = { api_base: "https://api-iam.intercom.io", app_id: "{YOUR_INTERCOM_APP_ID}" // Replace with your Intercom app ID. }; if (window.Intercom) { window.Intercom('reattach_activator'); window.Intercom('update', intercomSettings); } else { const intercomScript = document.createElement('script'); intercomScript.type = 'text/javascript'; intercomScript.async = true; intercomScript.src = 'https://widget.intercom.io/widget/{YOUR_INTERCOM_APP_ID}'; // Ensure this matches your Intercom app ID. intercomScript.onload = () => window.Intercom('update', intercomSettings); document.body.appendChild(intercomScript); } }, []); return null; // This component does not render anything visually. }; export default IntercomClientComponent;
- Replace `{YOUR_INTERCOM_APP_ID}` with your actual Intercom app ID.
Step 2: Integration within the layout.tsx
Now that your `IntercomClientComponent` is ready, it's time to integrate it into your project's layout. Here’s how you can do it:
- Open or create your layout.tsx file within the src/layouts directory.
- Add the IntercomClientComponent to your layout. Here’s an example layout implementing the component:
// src/layouts/RootLayout.tsx
import React from "react";
import Script from "next/script";
import IntercomClientComponent from "@/components/IntercomClientComponent"; // Adjust the path as necessary.
const RootLayout = ({children}: { children: React.ReactNode; }) => (
<html lang="en" className="h-full scroll-smooth">
<body className="relative h-full font-sans antialiased bg-white dark:bg-black text-black dark:text-white">
<main className="relative flex-col">
<div className="flex-grow flex-1 mt-20 min-h-screen">
{children}
</div>
</main>
<Script
strategy="afterInteractive"
id="intercom-settings"
dangerouslySetInnerHTML={{
__html: `
window.intercomSettings = {
api_base: "https://api-iam.intercom.io",
app_id: "{YOUR_INTERCOM_APP_ID}", // Ensure this matches your actual Intercom app ID.
};
`
}}
/>
<IntercomClientComponent/>
</body>
</html>
);
export default RootLayout;
Optimizations and Best Practices
Integrating scripts like Intercom's requires consideration around performance and security. In Next.js 14, leveraging the built-in `Script` component or dynamic imports with proper event handling ensures optimal load times without sacrificing user experience or security.
Common Issues and Troubleshooting
When integrating third-party services, issues can arise:
- Errors in the Intercom Event Handlers: Ensure that your component lifecycle management aligns with Intercom's API expectations.
- Script Loading Issues: Verify that your script tags are loading after interactive DOM elements to prevent render blocking.
Effective integration of Intercom within your Next.js 14 project not only elevates your user engagement but also leverages the state-of-the-art features offered by both platforms. This guide should serve as a comprehensive resource for integrating customer communication seamlessly into your digital project.
We encourage feedback and dialogue among our tech enthusiast community. Share your thoughts, experiences, or inquiries below. Happy coding!
Feedback and Community Contributions
We highly value the input and contributions from our developer community. If you have implemented Intercom in your Next.js projects and have insights, optimizations, or solutions that differ from this guide, please contribute to our repository. Community-driven advancements help us all to grow and improve our implementations. https://github.com/tantainnovative/intercom-next-js-14-guide
Future Directions
With the rapid evolution of web technologies, Next.js and Intercom will undoubtedly introduce new features and improvements. We aim to keep this guide updated with the latest best practices and encourage the community to participate in sharing updates and new techniques. Stay tuned for future revisions as we continue to explore more efficient, secure, and user-friendly integration methods.
Related Posts
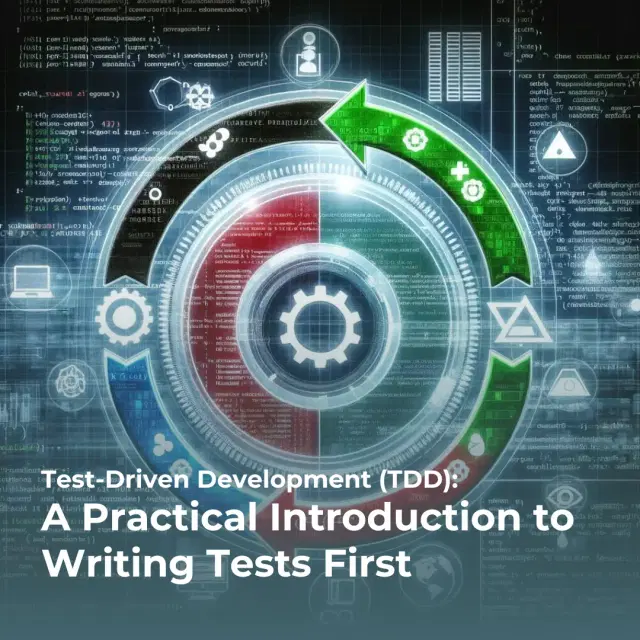
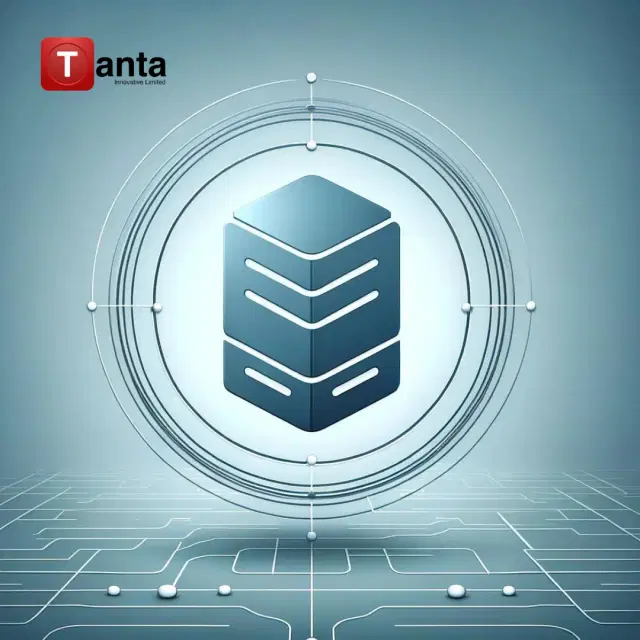
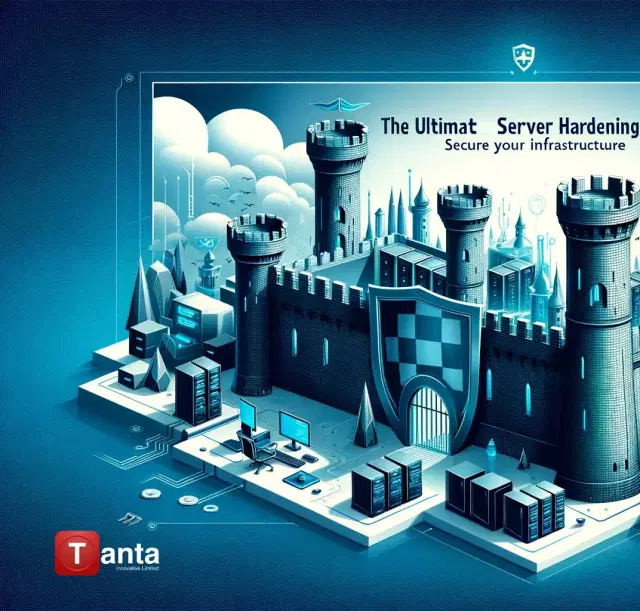